Linear search is a very simple search algorithm. In this type of search, a sequential search is made over all items one by one. Every item is checked and if a match is found then that particular item is returned, otherwise the search continues till the end of the data collection.
前言
线性搜索,就是从头找到尾,依次来看data[0]是否等于x,如果不是data[1],data[2],依次类推,一直找到最后一个。速度最慢,但是适用性最广。
线性搜索
线性搜索基本思想:
顺序查找也称为线形查找,属于无序查找算法。从数据结构线形表的一端开始,顺序扫描,依次将扫描到的结点关键字与给定值k相比较,若相等则表示查找成功;若扫描结束仍没有找到关键字等于k的结点,表示查找失败。
线性搜索解析:
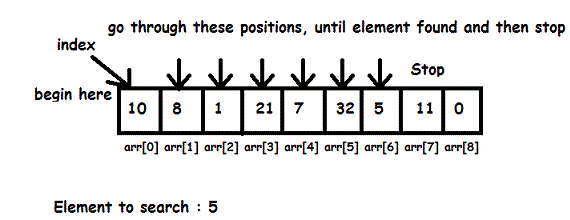
线性搜索动图:
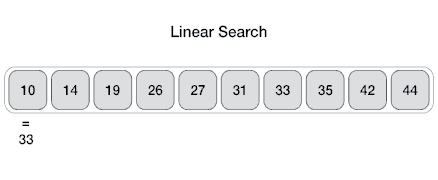
基数排序代码:
1 | /** |
延伸
顺序查找
顺序查找
顺序查找
韩顺平数据结构和算法
Data Structure and Algorithms Linear Search